Resources for KS3 Computing and GCSE Computer Science
These are free resources for the teaching of KS3 Computing and GCSE Computer Science. You can sequence them yourself (with the help of the curriculum concept map below) or see how I've incorporated them into a scheme of work. They are aimed at helping teachers to deliver KS3 Computing or GCSE Computer Science courses, but could also be used for self-study or revision. In addition to the detail, it's also worth having an idea of what Computing is. They were all produced by the creator of this site and are free to use under a Creative Commons BY-NC-SA licence. There is another section of the site that aims to crowd-source a set of quality, free, third-party Computing and Computer Science resources - these are categorised, searchable and you can contribute your own links.
The 2014 National Curriculum for England is less detailed than previous versions* - at Key Stage 3 it describes a common core for each subject that teachers are free to embellish and augment as they see fit, and at Key Stage 4 it just says that Computing should be taught but gives no further detail. Interestingly, the draft version included a tenth bullet point for KS3 Computing, "understand and use binary digits, such as to be able to convert between binary and decimal and perform simple binary addition", which seems to have been divided between Boolean logic and representation in the final version.
When planning a Computing curriculum, one question you could ask yourself is "What does it look like if someone is good at Computing?" Think about the things that such a person could do, make a list and sequence them into an appropriate order so that areas that depend on other topics come later in the course. The following is the list of topics that I chose - together with free resources to teach them. I've left the order up to you, so it's more a specification or programme of study than a scheme of work, but it represents what I would like students to know by the time they leave school and there's link to a suggested scheme of work below.
The list includes, and expands upon, those topics included in the National Curriculum for Computing. If students get a good grounding in the theory at Key Stage 3, you will find that there is actually very little left to cover for GCSE Computer Science, and you can concentrate on practising programming techniques and revising for the exam.
Don't just take my word for it - a Twitter/X user (to whom I have no connection) said of this site, "Everything you need to cover the GCSE Computer Science spec. Lots of interactive elements to engage students too. A complete resource website. Excellent!"
KS3 Computing Curriculum
There are no attainment targets in the new National Curriculum, so I have split the course into six sections that map approximately to the bullet points in the KS3 curriculum:
- Representation of Data - how different types of data are all stored as numbers inside the computer (and numbers are stored as binary)
- Maths for Computing - including number bases, Boolean logic and an introduction to the idea of graph theory
- Algorithms and Programming - including sorting, searching, Scratch, BASIC and Python
- Networking - including transmission and error-detection
- Information Systems - including spreadsheets, databases, and the use of HTML and CSS to create web-pages
- E-Safety
- Themes
- Examples, Revision, Scheme of Work and Assessment
Notice that I don't see "computational thinking" as a separate topic - it's more a side-effect of having sound knowledge and understanding of the other topics in the curriculum, and being able to link them together.
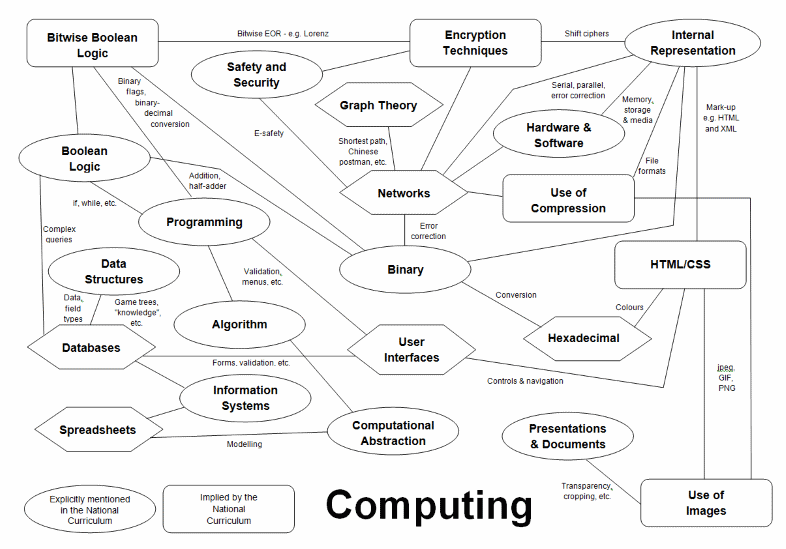
Some of these topics are of conceptual interest, while others, such as wireless networking, will be of practical use in the home or workplace. I have grouped them to facilitate assessment and reporting. Links are to pages within this site, PowerPoint shows to introduce topics to the class, or to resources and examples I have created in other sites such as Scratch or Python examples in Basthon. There are also supporting blogs and a YouTube channel.
Computing Curriculum Concept Map
One of the reasons that I prefer KS3 Computing to the old KS3 ICT curriculum is that the topics link to form a coherent whole, and my students appear to like that too. In Visible Learning, John Hattie identifies linking areas of the curriculum as one of the top ten most-effective teaching techniques, and empirical evidence suggests that this is something that teachers from a non-Computing background struggle to do. I have therefore also created a Computing curriculum concept map to show how these topics are related and what links them together.
Creating a Scheme of Work
Hattie also makes the point that buying, or using, someone else's scheme of work makes teachers less effective because they spend less time thinking about the curriculum as a whole, and that teachers and departments that create their own curriculum or scheme of work have more "impact". That is why the resources on this page are presented in no particular order - it is important that you think about how these ideas are related, and create a sequence that would best suit your own students. I have written an article to help you, explaining how to create a free computing course.
Topics and Resources
Before you start you might consider discussing what computer science is and what a computer scientist does.
Representation of Data
Note that the resources below were all made by me (unless otherwise stated). I've also created a crowd-sourced database of links to Computing resources on other sites - feel free to contribute your own resources.
- Internal representation of data, including...
- Binary for numbers (see also the Binary Breakout game)
- Text (also covered in the Character Functions section of Programming)
- Characters (i.e. symbols in typefaces/fonts)
- Images
- Vector v. Bitmap (see also Vectorise)
- Use of RGB and HSL to represent colours
- Bitmap formats (GIF, jpeg, PNG, etc.) - comparison of size, features, alpha, etc.
- Understand the importance of metadata
- Understand why it's important to know how images are stored (so that they can be manipulated)
- Sound
- Recording sounds - see the soundwave and samples and calculate the file size
- Sampling - see the effect or sampling rate and sample size
- Wave v. MIDI
- Wave formats - Ogg Vorbis, MP3, FLAC, etc.
- Data Structures (see also examples of their use in programming)
- Lists, queues, stacks, etc. (implementation using arrays in programming section?)
- Trees and traversal (see also Huffman Coding)
- Binary trees and storage in arrays
- Game trees and "knowledge"
- Storage (including cloud storage)
- Bits, bytes, etc.
- Properties of different storage media
- Error detection, RAID, etc.
- Security of files
- Collecting data...
- In a form to facilitate data processing
- Coding, granularity, etc.
- data, information and knowledge
- Calculating file sizes
- Compression - see interactive demo of RLE and Huffman Coding
- Demonstration of compression techniques including Run-Length Encoding and Huffman Coding
- Programmed examples: Run-Length Encoding, Huffman Coding, Dictionary Compression
- Stored Programs - why computers are different from calculators, the function of the CPU and the fetch-execute cycle (opcodes/instruction sets are covered in the representation presentation and here)
- the representation of data links the whole of Computing
Maths for Computing
- Number bases, including...
- Binary for numbers
- Binary Breakout (binary conversion game)
- Binary counter (look at the frequency with which the digits alternate)
- Range of binary values
- Powers of 2 / normalised floating point binary
- Binary arithmetic
- binary addition (interactive)
- binary fractions and negative numbers (interactive)
- decimals, negative and floating point numbers (presentation)
- Hexadecimal
- Binary for numbers
- Modular arithmetic (see also this article plus programming examples: Teams, Shuffle, Caesar-Shift, etc.)
- Boolean Logic and Bitwise
Boolean Logic (also Truth Tables, Operator Precedence and Duality)
- Boolean Logic (aka logic gates)
- Operator precedence
- De Morgan's Duals
- Logic circuits (including adder circuits
- Bitwise logic
- Binary flags (and shifting/masking)
- Adder circuits
- Logical Reasoning
- Introduction to paths and graphs
- Shortest paths
- Chinese Postman Problem
- Use of spreadsheets (or programming language?) to convert between denary and binary (see also this video)
- Scaling and reflection - e.g. random numbers, fitting things on the screen, bouncing
- Relationships - one-to-one, one-to-many, etc.
Algorithms and Programming
- Algorithms:
- Representation: flowcharts/pseudocode
- Examples: sorting (inc. sorting game (with demonstration)), merge sort, searching, mazes, drawing a circle, conversion to/from binary
- Interactive games to develop understanding of Bubble Sort, Merge Sort and Insertion Sort
- Efficiency (video on drawing circles)
- Recursion, e.g. flood fill
- Scratch
- Programming - general (see also the Programming section of the site):
- Programming (Just Basic):
- Introduction, PRINT and INPUT
- Variables
- Repetition with FOR, WHILE and UNTIL
- Decisions
- Select Case
- Random Numbers
- Arrays
- Text Functions
- Character Functions
- Numerical Functions & rounding
- Functions and procedures:
- Scope of variables
- Recursion
- By value and by reference
- Programmed examples from other areas of the curriculum
- Programming - Python (the same as the BASIC course, plus extra variable types, lists, etc.)
- Python presentations (zipped)
- Python examples (zipped)
- OCR Coding Challenges (with some possible solutions)
- Programmed examples from other areas of the curriculum (zipped)
- Python 3 YouTube playlist
- Programming - Visual Basic
- Forms, objects, properties and methods
- Extra variable types
- Visual Basic YouTube playlist
- Worked example
- What is Object-Oriented Programming?
When students are working on programming topics, I also sometimes give them logic puzzle starters.
Networking
- Networks (enhanced version for KS4)
- Definition
- Types
- LAN/WAN
- Client-server and peer-to-peer
- topologies
- protocols and layers
- encryption
- Uses of the internet
- Use of the Worldwide Web:
- Effective searching
- Assessing the quality of web-pages
- Privacy and personal safety
- E-Mail - POP/SMTP/IMAP
- Home networks:
- IP addresses and Ports (Ping to demonstrate)
- WiFi security
- Firewall
- DNS (Ping to demonstrate)
- Naming conventions - e.g. UNC, \\server1
- Internet connections: ADSL, FTTC, FTTH, etc.
- Transmission (parallel/serial) and error detection - parity, etc.
- Card Flip Magic (parity game) or Card Flip Magic (with digits) - click/tap to flip the cards, press a key or touch with two fingers to add parity bits; challenge a student to flip a card while you look away, it's the card in the row and column with the odd number of black cards (or 1s). Video demonstration of Card Flip Magic.
- Topologies, cabling, etc. (algorithm for least cable)
Information Systems
- Software - definition and examples, operating systems, applications and utilities, now including hosted applications and software as a service
- Spreadsheets
- Databases (Introduction to Databases)
- Fields and field types
- Queries and the use of Boolean logic in Queries
- Forms
- Reports
- Validation and verification (Validation and Verification presentation)
- Microsoft Access YouTube playlist
- Creating a Library System in Access YouTube playlist
- SQL Injection (and task)
- Relational databases and normalisation
- Use of compression and other techniques to make files smaller
- Programmed examples: Run-Length Encoding, Huffman Coding
- Introduction to HTML
- Creating web-pages (no use of wizards, etc. - students must understand everything that they make)
- Design considerations - mobile devices, availability of fonts (use of embedding?)
- No HTML styling - CSS only
- Use of selectors
- Use of :hover for menus, rollovers, etc.
- Use of DIVs - no tables for layout!
- Positioning and sizing - relative v. Absolute
- Use of images, transparency and translucency
- Forms and scripting
- Animation (using CSS keyframes rather than Flash)
- Creating Webpages with HTML and CSS YouTube playlist
- User interfaces
- Developments in Computing (e.g. moving from mainframes to PCs to networks and client-server) and their impact
- Project management: Gantt charts / Critical Path Analysis
- Systems for monitoring and control
E-Safety
- news stories on the cultural, ethical, legal and social impact of technology
- Does technology have an adverse effect on academic achievement? (also E-Safety - does technology impede learning blog)
- Cyber-Crime
- SQL Injection (with task)
- How Technology is Changing Our Brains
- Not all new technology is an improvement on older solutions
- How machine learning works and its impact (also AI - Why All the Fuss? blog)
Themes
Most of the points above relate to invidividual specification points. You can also improve understanding the identifying themes that run through these points:
There are also ideas that aren't explicitly mentioned in the specification but are really just combinations of things that are:
Examples
There are examples - particularly of the e-safety and cybersecurity elements of the course - in the news all of the time. The best examples are included on the Impact page, but for the latest examples look for the feeds on Bluesky or Facebook.
There are examples of programming techniques, and tasks and solutions, on the Learn to Program and OCR Coding Challenges pages. Finally, there are longer discussions of some topics (and relevant pedagogy, etc.) on the Computing and ICT in a Nutshell blog.
Videos
There are videos to support the teaching and revision of some of these concepts in the Computing and ICT in a Nutshell YouTube channel, as well as practical guides on other things such as the use of Microsoft Access, some aspects of LibreOffice, HTML, Scratch and Python.
Revision
There are plenty of external revision resources, such as those available in Computing resources database, but I have also created a limited number of revision resources:
- Connecting Wall (based on the BBC Only Connect quiz programme)
- Computing Worderama (a Word Mastermind-style word game using terms from the GCSE specification)
- Theory content on the Computing and ICT in a Nutshell YouTube channel
KS3 Computing Scheme of Work
There is a page to show the order that I teach these topics in the first year of my KS3 Computing course, but it is better for you to create your own scheme of work- here I describe how the curriculum map can be used to do that. You can create your own scheme of work and then source the individual lesson resources from elsewhere.
All of the links above are to my own resources, but I have also created a Computing resources database, to collect and collate high-quality third-party free Computing - look at the Links page.
Assessment of Computing
For assessment, I used a set of skill statements adapted from the CAS progress pathways document but I don't bother with the colours or numbering the levels - I record whether the student is sometimes successful at performing the named skill, or whether than can do it confidently and consistently. You can also read my thoughts on measuring progress in the Computing and ICT in a Nutshell blog.
Information Technology
If you're new to education in England, then you might be interested to see the 1995 National Curriculum for Information Technology.
Until 2014 the National Curriculum contained level descriptors to help teachers give a nationally-recognised description of a students' attainment. These were controversial for a number of reasons, but some schools have struggled to replace them.
Information Technology (IT) was renamed Information and Communication Technology (ICT) in 1998 and removed from the National Curriculum in 2013, a year before Computing was introduced. Whilst some ICT teachers complained about the "introduction" of programming, we can see that nearly twenty years before students were required to "develop, trial and refine sets of instructions to control events, demonstrating an awareness of the notions of efficiency and economy in framing these instructions".