Performance of Python
GCSE Computer Science requires you to know about compilers and interpreters and their relative merits. Most people think that there's a performance benefit in running a compiled language because the execution of compiled programs doesn't require any time for the translation stage - it's already been done. You might also be aware that, where speed of execution is important, certain languages are preferred - e.g. C++ is used for video games, not Python.
You might imagine that the differences between languages would be small, like comparing the performance or fuel economy of similar cars. You may also have seen league tables of the "greenest" programming languages (see below).
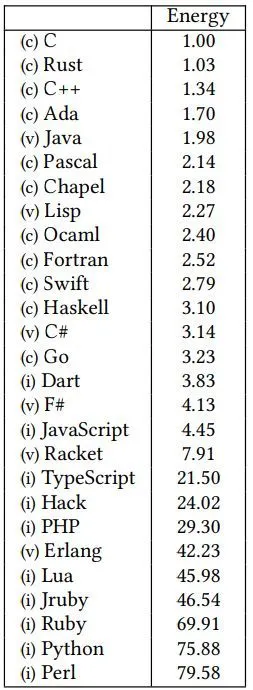
What does that mean? How are computer programs ranked in terms of environmental impact? The simple answer is that the amount of energy used is proportional to the amount of time required to run the program. If execution takes longer, then the computer runs for longer, consuming electricity as it goes. If a program requires more processing capacity than you have available, you might also need additional hardware.
Python often comes near the bottom of these tables, but just how slow is it? If you're reading this page then you probably use Python - but also have access to a web browser that can run JavaScript, so let's compare the two.
Example
Here's the Smallest Multiple task from the practice tasks page (and also Project Euler). We could use a brute force approach to test increasingly-large numbers to see whether they have all of the numbers as factors:

Using this method, the more numbers that are checked, the greater the amount of processing required. In fact, when I do this with my students I suggest that they test that their program finds the number in the example, and then they check numbers up to 15, rather than 20, so that they don't have to sit around waiting for the result.
Python
You may be able to come up with a better way of solving the problem, but I coded the same algorithm in Python and JavaScript. In fact, I started with the Python version and translated it, line by line, into JavaScript to ensure that algorithm was the same.
You can open the program in Basthon or you can open the source code and paste it into your favourite IDE. If you want to know how the code works, there's also a commented version without the timer.
When I run this program locally, using Python 3.13 in IDLE or Visual Studio Code on my three-year old computer, it takes just under a minute to find the result. Using Basthon in Firefox it takes over two minutes.
JavaScript
You don't need to download anything to run the JavaScript version - just click the Calculate button below. If you want to check that the code is comparable, just view the soure of this page and look at the smallest() function, which starts on line 50. Even if you're not familiar with JavaScript, you should be able to recognise that the program is basically the same (although it writes the answer to the page, rather than printing it).
Using Firefox on my three-year old computer, the answer appears in just less than a second. On my four-year old budget phone it takes just under 2.5s.
Does It Matter?
So you might think that the differences between programming languages are slight, but the Python version of the program takes about sixty times longer. That's like a family car taking ten minutes to reach 60mph, or doing 5mpg.
Whether this really makes a difference depends on what you're doing. If time is critical, then yes - obviously it does. In the past, for example, I have attempted some of the Advent of Code tasks in Python and I've had to leave them running overnight because they've taken so long. I also created a Sudoku solver program that takes seconds to run in the browser, but minutes to run when using Python.
On the other hand, I also wrote a blog, In Praise of Slowness, in which I said that it can be good to learn with something really slow because it gives you a greater appreciation of efficiency in your algorithms.